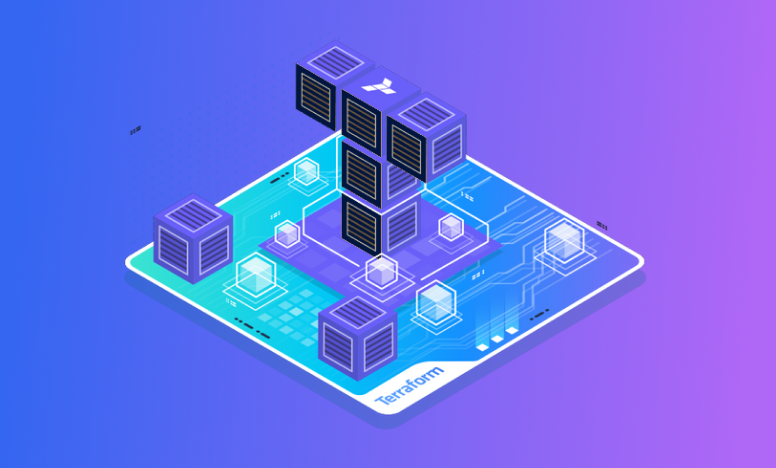
Key Terminologies
- Infrastructure as Code (IaaC): A methodology that allows developers to manage infrastructure resources using code, enabling consistency, scalability, version control, and automation.
- Terraform: A popular IaaC tool developed by HashiCorp that uses a declarative approach to manage infrastructure as code.
- Provider: A plugin in Terraform responsible for interacting with various cloud or infrastructure platforms (e.g., AWS, Azure, GCP).
- Resource: Specific infrastructure objects to create, update, or delete, representing components like EC2 instances, S3 buckets, or VPCs.
- Variable: Used to parameterize configurations and enable reusability of values across multiple resources or files.
- Data Source: Allows querying and retrieving information about existing resources, like EC2 instances or security groups.
- Output: Displays values or information after running terraform apply, useful for sharing critical data like IP addresses or resource IDs.
- Local Value: Defines intermediate values that can be used elsewhere in the configuration.
- Module: A collection of resources and configurations that can be reused across different parts of the infrastructure.
- Backend: Defines the configuration for storing Terraform state remotely (e.g., S3, Azure Storage).
- Provisioner: Executes scripts or commands on a resource after it is created.
- Resource Dependencies: Specifies explicit dependencies between resources using depends_on attribute.
- State File: Tracks the current state of the infrastructure managed by Terraform, allowing informed decisions during subsequent runs.
- Local Backend: Default backend that stores the state file in the current working directory (not recommended for production).
- Remote Backend: A secure option to store the state file in remote locations like S3, Azure Storage, or Google Cloud Storage.
Introduction
In the ever-evolving landscape of software development and cloud computing, Infrastructure as Code (IaaC) has emerged as a game-changer. IaaC allows developers and operations teams to manage and provision infrastructure resources through code, eliminating the need for manual configurations and ensuring consistency and scalability. In this blog, we will delve into the fundamentals of IaaC and explore one of its most popular tools, Terraform. So, fasten your seatbelts as we embark on a journey to demystify IaaC and unlock the power of Terraform.
What is Infrastructure as Code (IaaC)?
Infrastructure as Code (IaaC) is a methodology that enables developers to define and manage infrastructure resources using declarative or imperative code. By representing infrastructure components, such as servers, networks, and databases, as code, IaaC brings numerous benefits, including:
Consistency: Code-based infrastructure ensures that all environments are created and configured identically, reducing the risk of inconsistencies between development, testing, and production setups.
Scalability: IaaC facilitates easy scaling of infrastructure resources up or down based on demand, allowing systems to adapt to varying workloads efficiently.
Version Control: With IaaC, infrastructure configurations can be versioned, providing a historical record of changes and simplifying rollbacks and collaboration among team members.
Automation: By automating infrastructure provisioning, IaaC reduces manual intervention, which leads to faster and more reliable deployments.
Collaboration: Developers and operations teams can work together seamlessly, as infrastructure changes are visible and manageable in the same version control system used for code.
Introducing Terraform
Terraform, developed by HashiCorp, is one of the most popular IaaC tools in the market. It allows users to define infrastructure in a domain-specific language (HCL - HashiCorp Configuration Language) or JSON format. Terraform follows a declarative approach, where users specify the desired state of the infrastructure, and Terraform handles the provisioning and management.
Key Concepts of Terraform
Providers: In Terraform, providers are responsible for interacting with various cloud or infrastructure platforms (e.g., AWS, Azure, GCP, etc.). Each provider offers resources that can be managed through Terraform code.
Resources: Resources are the building blocks of infrastructure in Terraform. They represent the components you want to create and manage, such as virtual machines, networks, and storage.
State: Terraform keeps track of the current state of your infrastructure in a state file. This file contains information about the resources that Terraform manages and helps to plan and execute changes.
Configuration: Terraform configuration files, typically named main.tf, define the desired infrastructure state and the resources that need to be created or managed.
Plan and Apply: Before applying changes to the infrastructure, Terraform generates an execution plan (terraform plan), which shows what changes will be made. Once reviewed, you can apply the changes using terraform apply.
Getting Started with Terraform
Installation: Start by installing Terraform on your local machine by following the instructions provided on the official Terraform website.
Windows:
Download Terraform:
Go to the official Terraform website: https://www.terraform.io/downloads.html
Download the latest Windows 64-bit zip archive (it will have a filename like terraform_0.14.9_windows_amd64.zip, but the version number may be different).
Extract the downloaded zip archive:
Right-click the downloaded zip file and choose “Extract All.”
Select the destination folder where you want to extract Terraform (e.g., C:\terraform).
Add Terraform to the PATH environment variable:
Open the Start menu and search for “Environment Variables.”
Click “Edit the system environment variables.”
In the System Properties window, click the “Environment Variables” button.
In the “System Variables” section, find the “Path” variable and click “Edit.”
Click “New” and enter the path to the Terraform executable, which is the folder where you extracted Terraform in step 2 (e.g., C:\terraform).
Click “OK” to close all windows.
Verify the installation:
Open a new Command Prompt or PowerShell window.
Type terraform --version
and press Enter.
You should see the installed Terraform version displayed in the output.
macOS:
Install Homebrew (skip this step if you already have Homebrew):
Open Terminal (you can find it in the Applications/Utilities folder).
Paste the following command and press Enter:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
Install Terraform using Homebrew:
In Terminal, type the following command and press Enter:
brew tap hashicorp/tap
brew install hashicorp/tap/terraform
Verify the installation:
In Terminal, type terraform --version
and press Enter.
You should see the installed Terraform version displayed in the output.
Linux:
Download Terraform:
Go to the official Terraform website: https://www.terraform.io/downloads.html
Download the latest Linux 64-bit zip archive (it will have a filename like terraform_0.14.9_linux_amd64.zip, but the version number may be different).
Extract the downloaded zip archive:
Open a terminal.
Navigate to the directory where you downloaded Terraform (e.g., cd ~/Downloads).
Extract the zip archive using the following command (replace the filename with the one you downloaded):
unzip terraform_0.14.9_linux_amd64.zip
Move Terraform to the appropriate location:
Use the following command to move Terraform to /usr/local/bin:
sudo mv terraform /usr/local/bin/
Give executable permissions (if necessary):
If Terraform doesn’t have executable permissions, use the following command:
sudo chmod +x /usr/local/bin/terraform
Verify the installation:
Open a new terminal.
Type terraform --version
and press Enter.
You should see the installed Terraform version displayed in the output.
Now you can start using it to manage your infrastructure as code.
Provider Configuration
Choose a cloud provider (e.g., AWS, Azure) and configure the necessary credentials to allow Terraform to interact with the cloud platform.
AWS (Amazon Web Services):
Provider Configuration (in provider.tf):
provider "aws" {
region = "us-west-2" # Replace with your desired region
}
Configuring AWS Credentials:
Install AWS CLI and run the following command to configure your AWS access key ID and secret access key:
bash
aws configure
Example Terraform resource (EC2 instance in main.tf):
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0" # Replace with your desired AMI ID
instance_type = "t2.micro"
}
Azure (Microsoft Azure):
Provider Configuration (in provider.tf):
provider "azurerm" {
features {}
}
Configuring Azure Credentials:
Create an Azure Service Principal and obtain the subscription ID, client ID (Application ID), client secret (Authentication key), and tenant ID.
Example Terraform resource (Azure Virtual Machine in main.tf):
resource "azurerm_virtual_machine" "example" {
name = "example-vm"
location = "East US" # Replace with your desired location
resource_group_name = "example-rg"
network_interface_ids = [azurerm_network_interface.example.id]
vm_size = "Standard_DS1_v2"
delete_os_disk_on_termination = true
os_profile {
computer_name = "example-vm"
admin_username = "adminuser" # Replace with your desired username
admin_password = "Password123!" # Replace with your desired password
}
storage_image_reference {
publisher = "Canonical"
offer = "UbuntuServer"
sku = "18.04-LTS"
version = "latest"
}
storage_os_disk {
name = "example-osdisk"
caching = "ReadWrite"
create_option = "FromImage"
managed_disk_type = "Premium_LRS"
}
}
Google Cloud Platform (GCP):
Provider Configuration (in provider.tf):
provider "google" {
project = "my-gcp-project" # Replace with your GCP project ID
region = "us-central1" # Replace with your desired region
}
Configuring GCP Credentials:
Create a Service Account Key in GCP and obtain the JSON key file containing the credentials.
Example Terraform resource (GCP Compute Engine instance in main.tf):
resource "google_compute_instance" "example" {
name = "example-instance"
machine_type = "e2-micro"
zone = "us-central1-a" # Replace with your desired zone
boot_disk {
initialize_params {
image = "debian-cloud/debian-10"
}
}
network_interface {
network = "default"
}
}
Remember to keep your cloud provider credentials secure and avoid exposing them publicly. Terraform supports various authentication methods, and you can choose the one that suits your workflow best. Always follow the best practices for managing credentials and access control to maintain a secure cloud infrastructure.
Write Your First Configuration
Create a new directory for your Terraform project and write your first configuration file (main.tf). Define a simple resource, such as an AWS EC2 instance, and specify its attributes like region, instance_type, etc.
Provider:
A provider is a plugin that Terraform uses to interact with a specific cloud or service provider (e.g., AWS, Azure, Google Cloud, etc.).
Example:
provider "aws" {
region = "us-west-2"
}
Resource:
A resource is a specific infrastructure object that you want to create, update, or delete, such as an EC2 instance, S3 bucket, or VPC.
Example:
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
}
Variable:
A variable is used to parameterize your configuration, allowing you to reuse values across multiple resources or files.
Example:
variable "region" {
description = "AWS region where resources will be deployed."
default = "us-west-2"
}
Data Source:
A data source allows you to query and retrieve information about existing resources, such as EC2 instances or security groups.
Example:
data "aws_ami" "example" {
most_recent = true
owners = ["self"]
filter {
name = "name"
values = ["my-ami"]
}
}
Output:
An output allows you to display values or information after running terraform apply, useful for sharing critical information like IP addresses or resource IDs.
Example:
output "public_ip" {
value = aws_instance.example.public_ip
}
Local Value:
A local value is used to define intermediate values that can be used elsewhere in the configuration.
Example:
locals {
instance_count = 3
}
Module:
A module is a collection of resources and configurations that can be reused across different parts of your infrastructure.
Example:
module "vpc" {
source = "./vpc"
vpc_cidr = "10.0.0.0/16"
}
Backend:
A backend defines the configuration for storing Terraform state remotely (e.g., in S3, Azure Storage) instead of locally.
Example (AWS S3 Backend):
terraform {
backend "s3" {
bucket = "my-terraform-state-bucket"
key = "terraform.tfstate"
region = "us-west-2"
}
}
Provisioner:
A provisioner allows you to execute scripts or commands on a resource after it is created.
Example:
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
provisioner "remote-exec" {
inline = [
"echo 'Hello, Terraform'",
"sudo apt update && sudo apt install -y nginx",
]
}
}
Resource Dependencies:
Terraform automatically determines the dependencies between resources, but you can explicitly specify dependencies using depends_on attribute.
Example:
resource "aws_security_group" "web" {
name = "web-sg"
# other attributes
}
resource "aws_instance" "web_server" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
security_groups = [aws_security_group.web.id]
depends_on = [
aws_security_group.web
]
}
Initialize and Apply
Run terraform init to initialize your project and download the required provider plugins. Then, run terraform plan to generate an execution plan and preview the changes that Terraform will make to your infrastructure. Finally, run terraform apply to apply the changes and create the defined resources.
terraform init # Initialize the Terraform configuration.
terraform plan # Generate an execution plan.
terraform apply # Apply the changes to create resources.
It is essential to run terraform plan before terraform apply to review the changes and ensure that you understand what Terraform will do. This allows you to catch any potential issues or unintended changes before they are applied to your infrastructure. The terraform apply command can make significant changes to your cloud environment, so use it with caution and review the execution plan carefully.
Managing State
Understand the concept of state files and explore ways to store them securely. Terraform supports various backends like local, S3, and more.
Managing the state in Terraform is a crucial aspect of its operation. The state file keeps track of the resources that Terraform manages, the relationships between them, and their current state. It helps Terraform make informed decisions during subsequent runs, such as planning changes or updating resources. Storing state files securely is essential to maintain the integrity of your infrastructure and sensitive data.
Terraform supports various backends to store state files securely. Let’s explore some of the common backends and their benefits:
Local Backend:
The default backend is the local backend, where Terraform stores the state file in the current working directory by default. This option is not recommended for production use because it exposes the state file to potential risks, like accidental deletion or unauthorized access.
Example:
terraform {
backend "local" {}
}
Remote Backend (S3):
Using a remote backend, such as S3 in AWS, ensures that your state file is stored securely in a durable and scalable location. This prevents accidental loss of state and provides better collaboration in team environments.
Example (AWS S3 Backend):
terraform {
backend "s3" {
bucket = "my-terraform-state-bucket"
key = "terraform.tfstate"
region = "us-west-2"
}
}
Remote Backend (Azure Storage):
Similar to AWS S3, you can use Azure Storage as a remote backend if you are working with Azure cloud resources.
Example (Azure Storage Backend):
terraform {
backend "azurerm" {
storage_account_name = "mytfstatestorage"
container_name = "tfstatecontainer"
key = "terraform.tfstate"
}
}
Remote Backend (Google Cloud Storage):
For Google Cloud Platform (GCP) users, Google Cloud Storage can be used as a remote backend.
Example (Google Cloud Storage Backend):
terraform {
backend "gcs" {
bucket = "my-terraform-state-bucket"
prefix = "terraform/state"
}
}
In this blog, we’ve covered some of the key concepts of IaaC and introduced Terraform as a powerful tool for infrastructure management. However, there are a couple of other foundational concepts that are essential to know:
State Locking
When working with Terraform in a collaborative environment, state locking is critical to prevent concurrent modifications to the state file. State locking ensures that only one user or process can make changes to the state at a time, avoiding conflicts and maintaining data integrity. Terraform provides various backends that support state locking, enabling secure collaboration among team members.
Terraform Workspaces
As your infrastructure grows, managing multiple environments becomes essential. Terraform workspaces allow you to create multiple instances of the same infrastructure, enabling easy environment segregation. Each workspace maintains its own state file, making it convenient to switch between development, testing, and production environments without impacting others.
By incorporating state locking and utilizing Terraform workspaces, you can confidently scale your infrastructure management and streamline collaboration in team environments.
Secure State Management
Secure State Management in IaC is crucial for maintaining the integrity, availability, and confidentiality of the provisioned infrastructure. By integrating security practices throughout the IaC development and deployment lifecycle, organizations can significantly reduce the risks associated with managing their infrastructure through code.
Best Practices for Secure State Management:
- Always use a remote backend for production environments to prevent accidental loss of state data.
- Ensure that access to the state storage bucket/container is properly restricted with appropriate IAM (Identity and Access Management) policies or permissions.
- Use versioning in your storage backend to keep track of state changes and roll back if necessary.
- Encrypt the state file at rest to protect sensitive information.
- Implement role-based access controls (RBAC) to limit who can access and modify the state file.
- Remember, the state file contains sensitive data like resource IDs and credentials. Keeping it secure is vital to maintaining the integrity of your infrastructure. Choose the backend that aligns with your cloud provider and security requirements, and follow the recommended best practices to store your state files securely.
Conclusion
Infrastructure as Code and Terraform have revolutionized the way we manage and deploy infrastructure resources. By representing infrastructure configurations as code, we gain consistency, scalability, and automation, making our systems more robust and adaptable to changes.
Armed with this foundational knowledge, you can now start your IaaC journey and unlock the true potential of automated infrastructure management with Terraform! Happy coding!