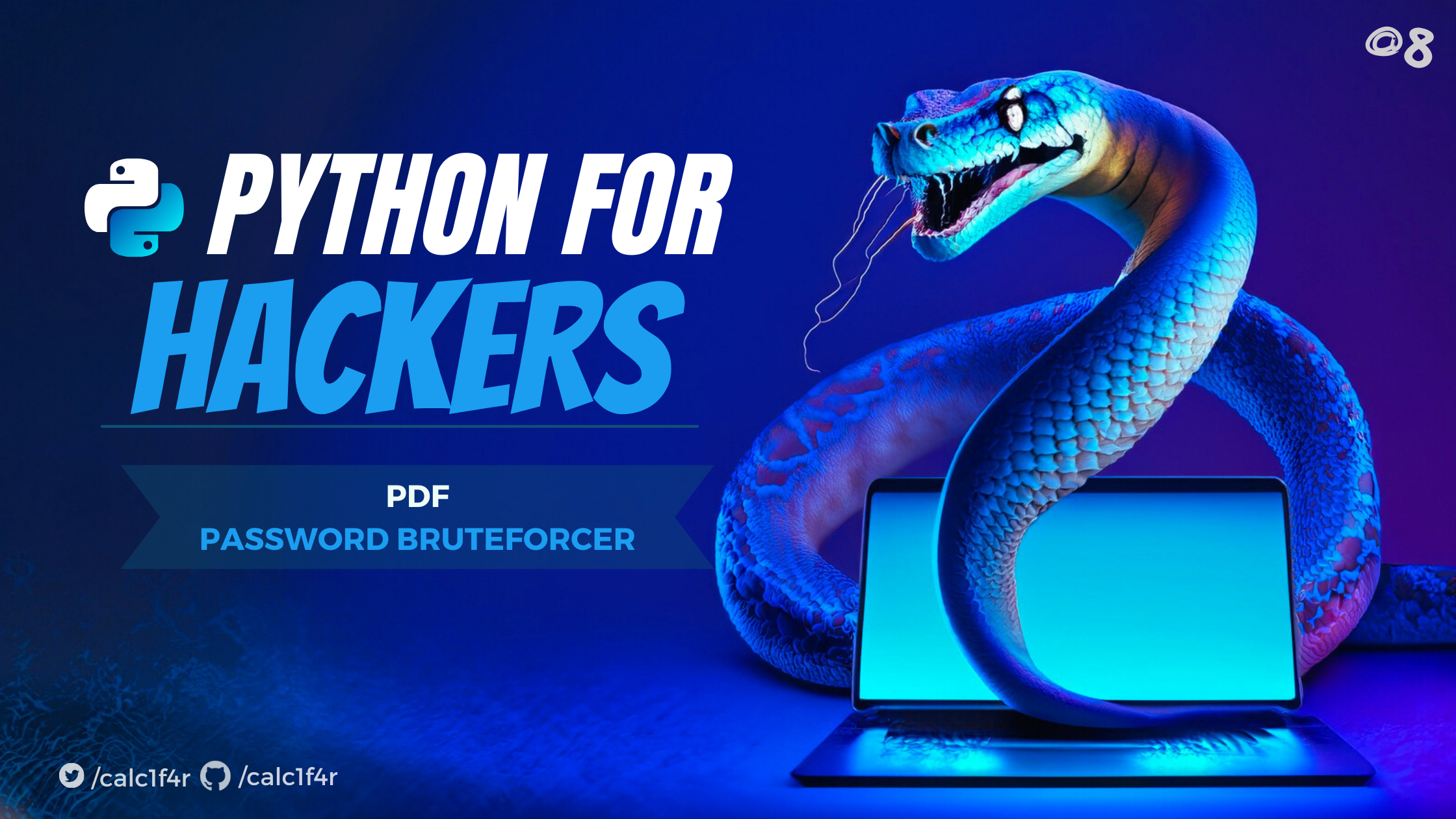
Hello Folks😗,
Welcome to the eighth blog of our “Python for Hackers” blog series. Today, we’re building a very easy to make tool which is Password Protected PDF file Bruteforcer with Python , which will help us Bruteforce every password file using a rainbow table attack.
🫐 Building the tool
🛠 Importing all the necessary modules in our file
from PyPDF2 import PdfFileReader,PdfFileWriter
from os import path
from sys import exit
from termcolor import colored
PyPDF2 is a free and open-source Python library for working with PDF files.
Coding out code outside functions
if __name__=="__main__":
pdfFile=input("[+] Enter the location of Password Protected File Pdf : ")
if not path.exists(pdfFile):
print(colored("[-] Please specify the location of Pdf file accurately ",'red'))
exit(-1)
wordlistfile=input("[+] Enter the location of password wordlist file: ")
if not path.exists(wordlistfile):
print(colored("[-] Please specify the location of wordlist file accurately ",'red'))
exit(-1)
password=bruteforcepdf(pdfFile,wordlistfile)
if password:
decrypt_pdf(pdfFile,f"decrypted-{path.basename(pdfFile)}",password)
else:
print(colored("[-] Password was not Found :",'red'))
Let’s understand the code
- Firstly we are checking for the namespace , if it is
__main__
, then we proceed further.
- Then we ask the user for location of the pdf file, and using path module which is present in the OS library we check if the pdf file at the given location exists or not and if it doesn’t exist we just simply exit out of the program.
- Then we prompt the user to give us a wordlist file and the same way as above , we check if the wordlist file exists or not.
- Then we call the main function responsible for Brute Forcing with arguments such as location of pdf file and location of wordlist file, and we are assigning the value it is returning to the password file.
- And the function has been executed, if we get a password value in return , we call another function called
decrypt_pdf
with arguments as location of the encrypted file, a custom string for decrypted file and the location of the wordlist file.
- If not we prompt the user that the password has not been found.
Coding out the bruteforcepdf function
def bruteforcepdf(pdfFile,wordlistfile):
"""Function to bruteforce pdf file"""
passwords=[]
with open(wordlistfile,'r') as f:
for password in f.readlines():
passwords.append(password.strip())
reader=PdfFileReader(pdfFile)
for password in passwords:
if str(reader.decrypt(password))=='PasswordType.OWNER_PASSWORD':
print(colored(f"[+] Password Found : {password}",'green',attrs=['bold']))
return password
Let’s understand various elements :
- We firstly declare a variable called passwords with an empty list.
- The we open the wordlist file and for every word after striping the trailing whitespaces from it, we just append the word to the passwords list.
- Then we create a reader object form the
PDFfileReader
class with the pdf file location as the argument.
- We loop over every password in passwords list , and we execute the function
reader.decrypt
with our password , if the string after executing the function is PasswordType.OWNER_PASSWORD
, which is the string the reader.decrypt
gives out , if the decryption is successful.
- if the condition is true , if print the password has been found and return the original password.
Coding out the decrypt_pdf
function
def decrypt_pdf(encypted_file,decrypted_file,password):
with open(encypted_file,'rb') as encryptedFile, open(decrypted_file,'wb') as decrypteFile:
reader=PdfFileReader(encryptedFile)
if reader.is_encrypted:
reader.decrypt(password)
writer=PdfFileWriter()
for i in range(reader.getNumPages()):
writer.addPage(reader.getPage(i))
writer.write(decrypted_file)
print(colored(f"File Has been Succcessfully decrypted and saved at : {decrypted_file}",'cyan'))
- Firstly we open both the files Encrypted file as
encryptedFile
and also decrypted file as the decrypteFile
.
- Then for the encrypted file we have defined a reader object derived from the The
PdfFileReader
class.
- Then we check if the file is encrypted , if yes we run the
reader.decrypt
method on the file.
- We define a writer object using
PdfFileWriter()
class.
- Then we loop over the range of number of files in the
encryptedFile
pdf.
- Then in the writer object we add the page after extracting from the reader object/
- Then after adding all the pages, we write the file with the name as the decrypted_file value.
- At last we print the file has been decrypted and saved at the location.