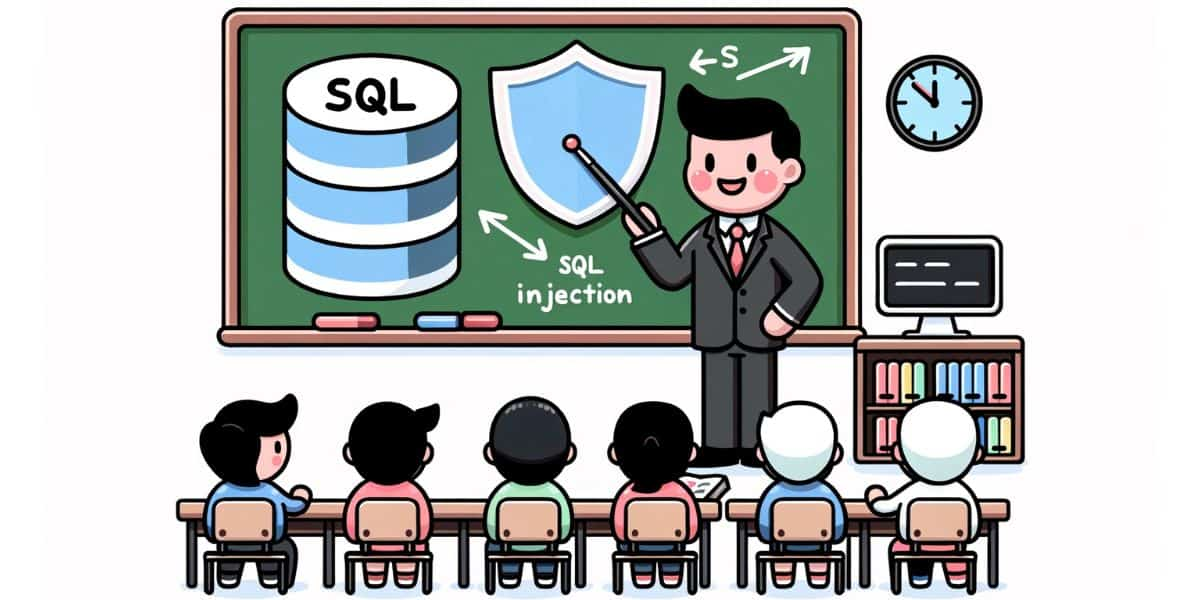
What is SQL Injection?
SQL Injection (SQLi) is one of the most common and dangerous vulnerabilities found in web applications. It’s an attack that allows hackers to manipulate SQL queries made by the application to interact with a database. By injecting malicious SQL code into a query, attackers can access sensitive data, modify the database, or even execute administrative operations.
Why is it Dangerous?
SQL Injection can lead to:
DataTheft: Attackers can exfiltrate sensitive information like usernames, passwords, credit card details, etc.
Data Loss/Manipulation: Hackers can delete or alter data, affecting application functionality.
Authentication Bypass: SQLi can bypass login forms, granting unauthorized access.
Privilege Escalation: Gaining administrator access through low-level permissions.
Remote Code Execution: In rare cases, attackers can gain full control over the server.
How SQL Queries Work
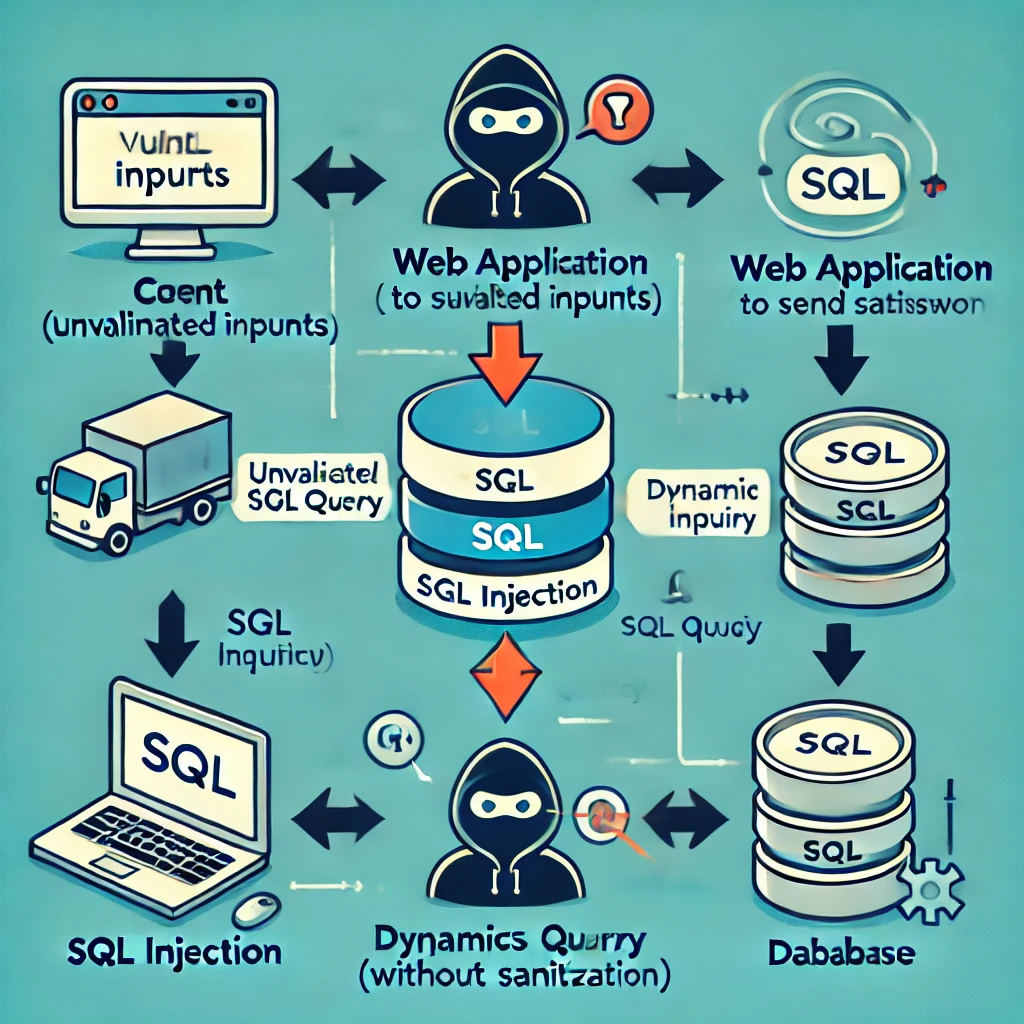
Client (Attacker):
A user or an attacker sitting at their machine, interacting with a web application through a browser.
Sends inputs (like login credentials) to the web application.
Web Application:
The vulnerable website where the user inputs are processed.
Receives the form data (like username and password), and this input is sent to the application’s backend.
In this case, the web application doesn’t validate or sanitize the input, making it susceptible to SQL Injection.
SQL Query:
The SQL query is dynamically constructed using the input data.
The vulnerable query is shown here without proper sanitization or parameterization.
Example :
SELECT * FROM users WHERE username = 'admin' AND password = 'password123';
This query asks the database to return all records from the users table where the username is ‘admin’ and the password is ‘password123’.
In a secure application, these values should be sanitized or parameterized. However, in vulnerable applications, user inputs are directly injected into the SQL query without validation.
Let’s say the application’s login page accepts user inputs for username and password, and sends them directly into the query:
SELECT * FROM users WHERE username = 'admin' --' AND password = '';
If a user enters admin’ – in the username field, and nothing in the password field, the resulting query becomes:
SELECT * FROM users WHERE username = 'admin' --' AND password = '';
The --
is a SQL comment, so everything after it is ignored. The query now checks if the username is admin
, but it doesn’t verify the password. As a result, the attacker gains access to the admin account without needing the password.
Types of SQL Injection
SQL Injection can be categorized into several types:
Classic SQL Injection: Direct injection of malicious SQL code.
Blind SQL Injection: Exploiting the database without any visible output. Instead of seeing results, attackers infer information based on the application’s behavior (e.g., delays, errors).
Error-based SQL Injection: Exploiting error messages to gather information about the database.
Time-based Blind SQL Injection: Using time delays to infer whether a query is successful or not, typically when no direct output is returned.
Union-based SQL Injection: Extracting data by appending a UNION SELECT query.
Conclusion
In this first part, we’ve covered the basic concepts of SQL Injection: what it is, why it’s dangerous, and how simple injections work. As we continue, the next parts will dive into blind SQL injection, advanced payload crafting, and methods to exploit SQL Injection in real-world applications.
Stay tuned for Part 2, where we’ll explore blind SQL injection techniques and how attackers gather data without visible outputs.
SQL Injection Payloads Used in Part 1
' OR '1'='1';
' UNION SELECT null, version(), database(), user() -- ;
' OR 1=1--